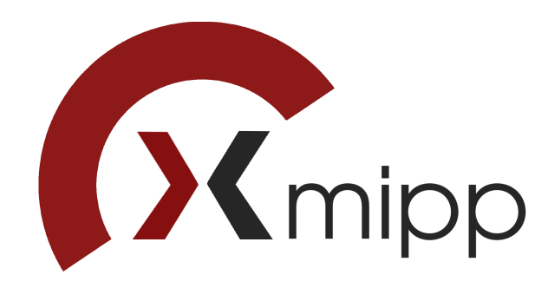 |
Xmipp
v3.23.11-Nereus
|
Go to the documentation of this file. 26 #ifndef CORE_XMIPP_MACROS_H 27 #define CORE_XMIPP_MACROS_H 33 #define MAXDOUBLE DBL_MAX 34 #define MINDOUBLE DBL_MIN 42 #define MINFLOAT -1e30 52 #define ISNAN(x) ((x)!=(x)) 54 #define ISNAN(x) isnan(x) 59 #define ISINF(x) (!ISNAN (x) && ISNAN ((x) - (x))) 61 #define ISINF(x) isinf(x) 64 #define ISNAN(x) std::isnan(x) 65 #define ISINF(x) std::isinf(x) 97 #define PI 3.14159265358979323846 104 #define HALFPI 1.57079632679489661923 111 #define TWOPI 6.283185307179586 119 #define XMIPP_EQUAL_ACCURACY 1e-6 122 #define XMIPP_EQUAL_REAL(x, y) ((fabs((x) - (y))) < XMIPP_EQUAL_ACCURACY) 123 #define XMIPP_EQUAL_ZERO(x) (ABS(x) < XMIPP_EQUAL_ACCURACY) 124 #define XMIPP_RANGE_INSIDE(x,min,max) ((x) >= (min) - XMIPP_EQUAL_ACCURACY && \ 125 (x) < (max) + XMIPP_EQUAL_ACCURACY) 126 #define XMIPP_RANGE_OUTSIDE(x,min,max) ((x) < (min) - XMIPP_EQUAL_ACCURACY || \ 127 (x) >= (max) + XMIPP_EQUAL_ACCURACY) 128 #define XMIPP_RANGE_OUTSIDE_FAST(x,min,max) ((x) < (min) || (x) >= (max)) 142 #define ABS(x) (((x) >= 0) ? (x) : (-(x))) 155 #define SGN(x) (((x) >= 0) ? 1 : -1) 169 #define SGN0(x) (((x) >= 0) ? (((x) == 0) ? 0:1) : -1) 181 #define XMIPP_MIN(x, y) (((x) >= (y)) ? (y) : (x)) 193 #define XMIPP_MAX(x,y) (((x)>=(y))?(x):(y)) 210 #define ROUND(x) (((x) > 0) ? (int)((x) + 0.5) : (int)((x) - 0.5)) 225 #define CEIL(x) (((x) == (int)(x)) ? (int)(x):(((x) > 0) ? (int)((x) + 1) : \ 240 #define FLOOR(x) (((x) == (int)(x)) ? (int)(x):(((x) > 0) ? (int)(x) : \ 247 #define FRACTION(x) ((x) - (int)(x)) 260 #define CLIP(x, x0, xF) (((x) < (x0)) ? (x0) : (((x) > (xF)) ? (xF) : (x))) 272 #define intWRAP(x, x0, xF) (((x) >= (x0) && (x) <= (xF)) ? (x) : ((x) < (x0)) \ 273 ? ((x) - (int)(((x) - (x0) + 1) / ((xF) - (x0) + 1) - 1) * \ 274 ((xF) - (x0) + 1)) : ((x) - (int)(((x) - (xF) - 1) / ((xF) - (x0) + 1) \ 275 + 1) * ((xF) - (x0) + 1))) 280 #define fastIntWRAP(y, x, x0, xF) \ 282 if (((x) >= (x0) && (x) <= (xF))) \ 286 int range=(xF) - (x0) + 1; \ 288 y=((x) - (int)(((x) - (x0) + 1) / range - 1) * range); \ 290 y=((x) - (int)(((x) - (xF) - 1) / range + 1) * range); \ 304 #define realWRAP(x, x0, xF) ((x) - floor(((x) - (x0)) / ((xF) - (x0))) * ((xF) - (x0))) 312 #define DEG2RAD(d) ((d) * PI / 180) 320 #define RAD2DEG(r) ((r) * 180 / PI) 329 #define COSD(x) cos(PI * (x) / 180.) 338 #define ACOSD(x) acos((x)) * 180. / PI 347 #define SIND(x) sin(PI * (x) / 180.) 356 #define ASIND(x) asin((x)) * 180. / PI 362 #define SINC(x) (((x) < 0.0001 && (x) > -0.0001) ? 1 : sin(PI * (x)) \ 374 #define NEXT_POWER_OF_2(x) pow(2, ceil(log((double) x) / log(2.0)-XMIPP_EQUAL_ACCURACY) ) 381 #define LIN_INTERP(a, l, h) ((l) + ((h) - (l)) * (a)) 387 #define XOR(a, b) (((a) && !(b)) || (!(a) && (b))) 394 #define SPEED_UP_temps0 \ 398 #define SPEED_UP_temps01 \ 403 #define SPEED_UP_temps012 \ 408 #define SPEED_UP_tempsInt \ 409 int ispduptmp0, ispduptmp1, ispduptmp2, \ 410 ispduptmp3, ispduptmp4, ispduptmp5; 413 #define SPEED_UP_tempsDouble \ 415 double spduptmp3, spduptmp4, spduptmp5, \ 416 spduptmp6, spduptmp7, spduptmp8; \ 419 #define SPEED_UP_temps \ 420 SPEED_UP_tempsDouble; \ 428 #define SWAP(a, b, tmp) {\ 439 #define FIRST_XMIPP_INDEX(size) -(int)((float) (size) / 2.0) 448 #define LAST_XMIPP_INDEX(size) FIRST_XMIPP_INDEX(size) + (size) - 1 458 #define SUM_INIT(var, value) if (first_time) var = (value); else var += (value); 459 #define SUM_INIT_COND(var, value, cond) if (cond) var = (value); else var += (value); 465 # define XMIPP_ASSUME_ALIGNED(ptr, alignment) (__builtin_assume_aligned(ptr, alignment)) 467 # define XMIPP_ASSUME_ALIGNED(ptr, alignment) (ptr)